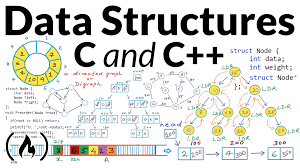
C/C++ and Data Structure
Price
NA
Duration
NA
Modules
C Programming Modules
Introduction to C Language
History, features, structure of a C program
Data Types, Variables, and Operators
Primitive types, constants, expressions
Control Flow Statements
If-else, loops, switch-case
Functions and Recursion
Modular programming, function calls
Arrays and Strings
One/two-dimensional arrays, string handling
Pointers and Memory Management
Pointer arithmetic, dynamic memory (malloc, free)
Structures and Unions
Defining, accessing, and nested structures
File Handling in C
Reading/writing files, file pointers
C++ Programming Modules
Introduction to C++ and OOP Concepts
Differences from C, basics of OOP
Classes and Objects
Access specifiers, constructors/destructors
Inheritance and Polymorphism
Types of inheritance, function overloading/overriding
Encapsulation and Abstraction
Interface design and data hiding
Operator Overloading and Templates
Function/operator templates and generic programming
STL (Standard Template Library)
Vectors, lists, maps, iterators
Exception Handling
Try, catch, throw, custom exceptions
File I/O in C++
fstream, ofstream, ifstream usage
Data Structures Modules
Introduction to Data Structures
Types, complexity analysis (Big O Notation)
Arrays and Linked Lists
Singly, doubly, and circular linked lists
Stacks and Queues
Implementation using arrays and linked lists
Trees and Binary Trees
Traversals, BST, AVL Trees
Heaps and Priority Queues
Max/min heap operations
Hashing and Hash Tables
Collision handling, hash functions
Graphs
Representation, BFS, DFS, shortest path (Dijkstra)
Sorting and Searching Algorithms
Bubble, merge, quick sort; linear/binary search